网易云音乐在近期与重庆独立嘻哈厂牌GOSH达成独家战略合作!歌单中为全部独家授权资源,一起来听最纯正的西南方言说唱吧!
文章源自两天的博客-https://2days.org/21291.html
文章源自两天的博客-https://2days.org/21291.html
文章源自两天的博客-https://2days.org/21291.html
文章源自两天的博客-https://2days.org/21291.html 文章源自两天的博客-https://2days.org/21291.html
本站文章大部分始于原创,用于个人学习记录,可能对您有所帮助,仅供参考!
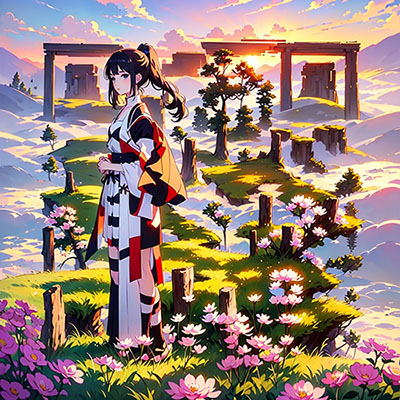
312379857
←QQ扫一扫添加QQ好友
版权声明:本站原创文章转载请注明文章出处及链接,谢谢合作!
评论